1. React의 LifeCycle
-
초기구성 : React 컴포넌트 객체가 DOM에 실제로 삽입되기 전까지의 과정을 마운팅(Mounting)이라고 하는데, 마운팅 시 아래의 LifeCycle 함수들이 순차적으로 수행된다.
-
constructor()
-
componentWillMount()
-
render()
-
componentDidMount()
-
컴포넌트가 모두 구성된 직후인 componentDidMount() 함수에서 데이터를 가져오는 API 호출을 수행하면 효과적이다.
-
2. API 호출 예제
2-1. fatch API를 사용해 서버로부터 데이터를 가져와 화면에 표출하는 예제
위와 같은 React LifeCycle의 개념을 활용해 API를 호출하는 예제를 작성해보자.
예제에서는 javascript ES6에서 지원하는 fetch API를 사용하여 서버와 통신을 할 것이다.
fetch함수는 클라이언트 상에서 특정 서버에 접속하고자할때 사용하는 함수이다.
fetch 완료 후에 응답을 처리할때는 then 함수를 이용한다.
-
fetch API 사용 예
//서버로부터 받은 응답을 json 형태로 콘솔에 출력 fetch('https://jsonplaceholder.typicode.com/todos/1') .then(response => response.json()) .then(json => console.log(json))
테스트 json 데이터를 가져오기 위해서 가짜(Fake) 온라인 REST API 사이트인 JSON Placeholder 사이트(jsonplaceholder.typicode.com/)를 이용했다.
[html]
<div id="root"></div>
[js]
class ApiExample extends React.Component {
constructor(props) {
super(props);
this.state = {
data: ''
}
}
callApi = () => {
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then(res => res.json())
.then(json => {
this.setState({
data: json.title
})
})
}
//특정한 api를 호출할때는 componentDidMount함수에서 호출!
componentDidMount() {
this.callApi();
}
//class형 컴포넌트는 render함수를 가지고 있음
//render함수가 먼저 수행된 이후에 componentDidMount를 호출. 따라서 초기에 api를 통해 데이터를 가져오기 전 this.state.data 값이 없을 경우를 고려해 render 함수를 작성
render() {
return (
<h3>{this.state.data ? this.state.data : '데이터 불러오는 중'}</h3>
)
}
}
ReactDOM.render(
<ApiExample />,
document.getElementById('root')
);
-
위 예제와 같이 컴포넌트가 렌더링 된 이후 호출되는 componentDidMount함수에서 데이터를 가져오는 api호출을 수행한다.
-
render 함수가 먼저 수행된 이후에 componentDidMount 함수를 호출되기때문에 초기에 api를 통해 데이터를 가져오기 전 this.state.data 값이 없을 경우를 고려해 render함수를 작성해야한다
-
초기에 api로 데이터를 가져오기전에는 this.state.data 가 없기때문에 '데이터 불러오는 중' 으로 화면에 표출되다가 이후 api를 통해 응답 json데이터를 가져온 후에는 응답 json데이터의 title이 표출된다.
[실행결과]
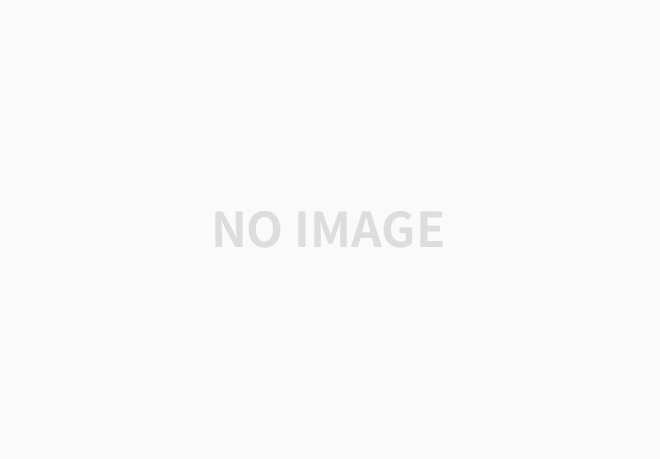
참조 강좌 및 블로그 출처
YouTube 동빈나 [React JS 이론부터 실전까지] 강좌, ndb796.tistory.com/227?category=1029507 [안경잡이개발자]
'Study > React' 카테고리의 다른 글
[React] 3. Component의 Props 와 State (0) | 2020.10.17 |
---|---|
[React] 2. React에서 JSX란? (0) | 2020.10.12 |
[React] 1. React 개요 및 코드펜(Codepen)을 이용한 React 개발환경 구축 (0) | 2020.10.11 |